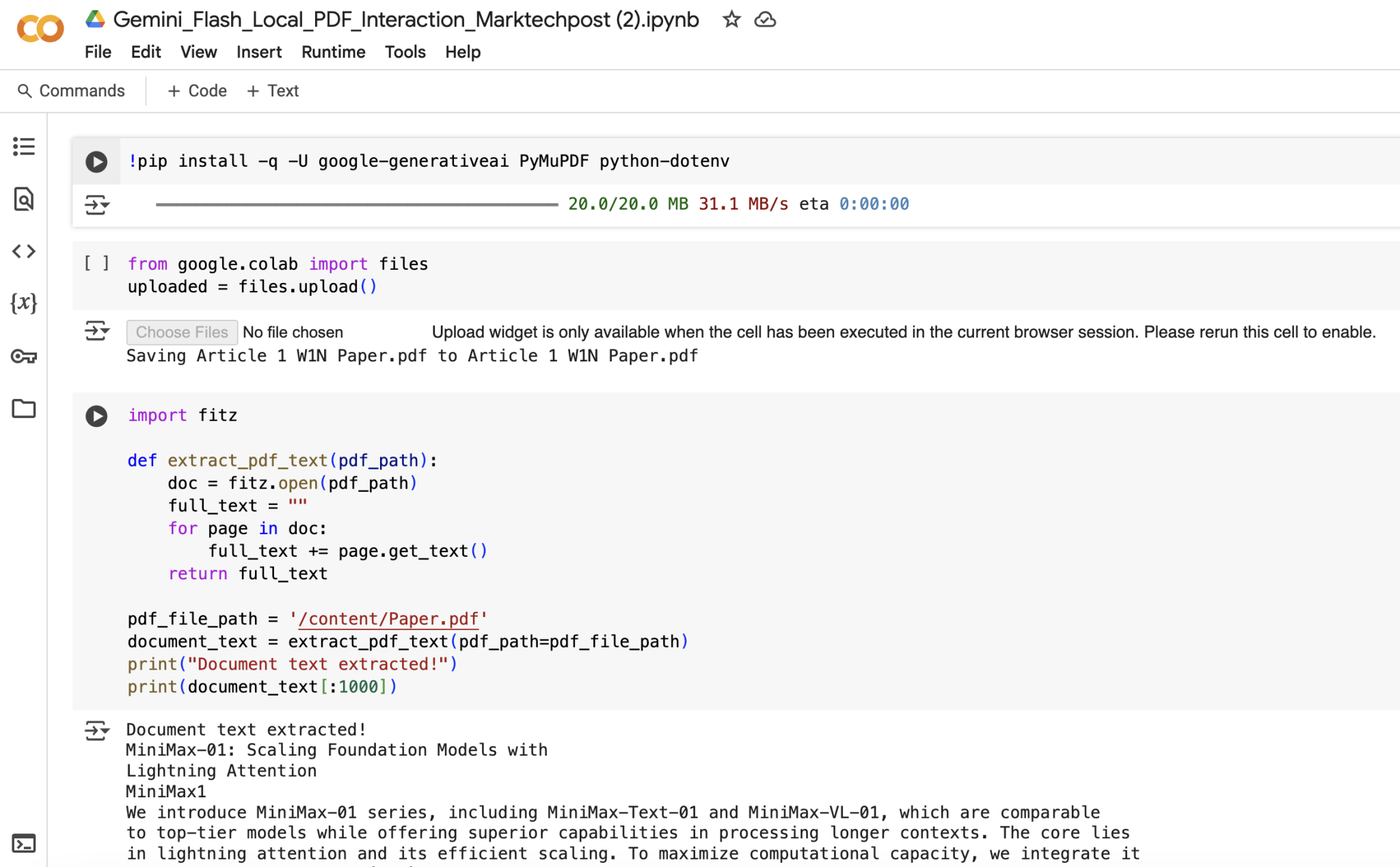
Building an AI-Powered PDF Interaction System
This tutorial outlines the steps to create an AI-driven PDF interaction system using Google Colab, Gemini Flash 1.5, PyMuPDF, and the Google Generative AI API. By utilizing these technologies, users can upload a PDF, extract its text, and ask questions to receive intelligent responses.
Step 1: Install Required Dependencies
Begin by installing the necessary libraries:
!pip install -q -U google-generativeai PyMuPDF python-dotenv
These libraries facilitate natural language interactions and efficient text extraction from PDFs.
Step 2: Upload PDF Files
Use the following code to upload files from your local device:
from google.colab import files uploaded = files.upload()
This allows you to select and upload a PDF file for processing.
Step 3: Extract Text from PDF
Utilize PyMuPDF to extract text from the uploaded PDF:
import fitz def extract_pdf_text(pdf_path): doc = fitz.open(pdf_path) full_text = "" for page in doc: full_text += page.get_text() return full_text pdf_file_path = '/content/Paper.pdf' document_text = extract_pdf_text(pdf_path=pdf_file_path) print("Document text extracted!") print(document_text[:1000])
This function reads the PDF and retrieves its text content, enabling further analysis.
Step 4: Set Up the Google API Key
Store your Google API key securely as an environment variable:
import os os.environ["GOOGLE_API_KEY"] = 'Use your own API key here'
This key allows access to the Google Generative AI services.
Step 5: Query the AI Model
Configure and query the Gemini Flash model:
import google.generativeai as genai genai.configure(api_key=os.environ["GOOGLE_API_KEY"]) model_name = "models/gemini-1.5-flash-001" def query_gemini_flash(question, context): model = genai.GenerativeModel(model_name=model_name) prompt = f""" Context: {context[:20000]} Question: {question} Answer: """ response = model.generate_content(prompt) return response.text pdf_text = extract_pdf_text("/content/Paper.pdf") question = "Summarize the key findings of this document." answer = query_gemini_flash(question, pdf_text) print("Gemini Flash Answer:") print(answer)
This setup enables automated summarization and intelligent question answering from the PDF.
Conclusion
By following this tutorial, you have built an interactive PDF interaction system in Google Colab. This solution simplifies information extraction and querying from PDFs, leveraging advanced AI models.
Further Engagement
Explore how AI can transform your business processes. Identify automation opportunities and key performance indicators to measure the impact of your AI initiatives. Start small, gather data, and gradually expand your AI applications.
For assistance in managing AI in your business, contact us at hello@itinai.ru or reach out via Telegram, X, or LinkedIn.