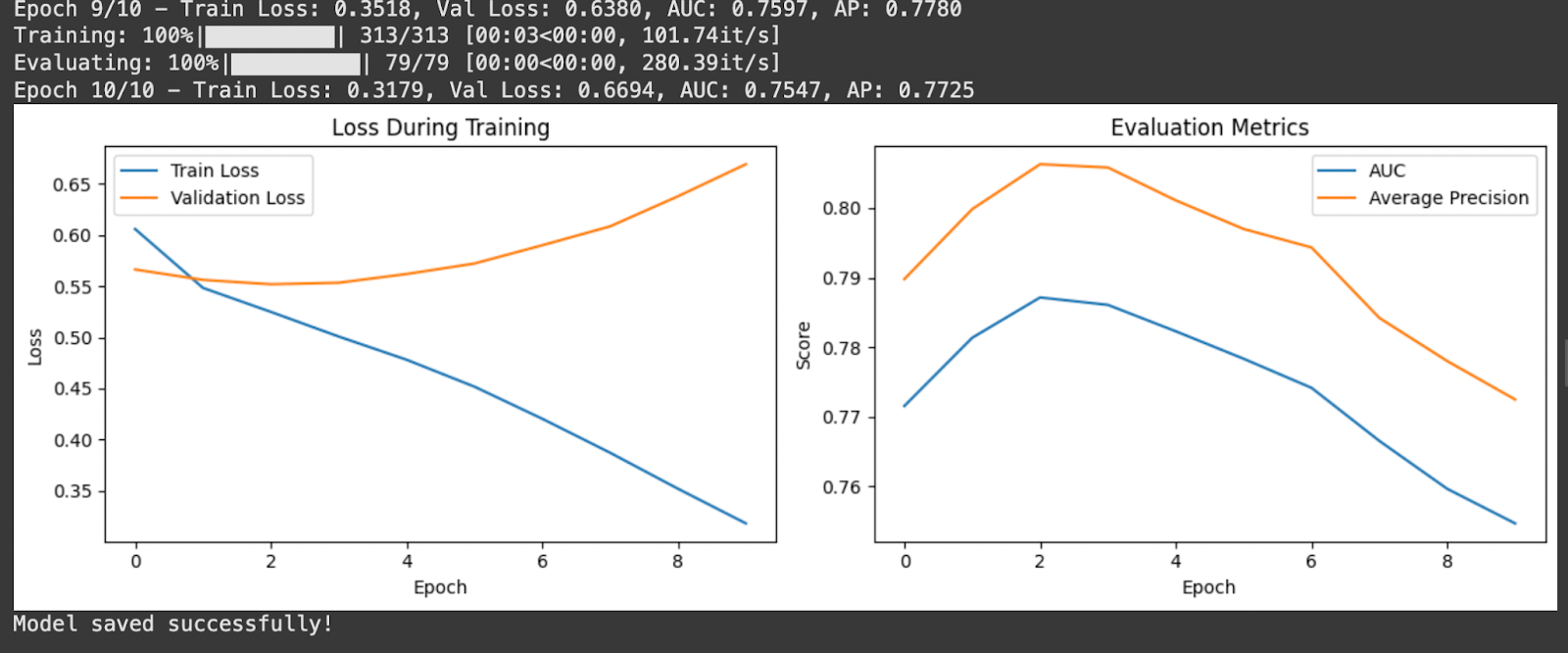
Building a Neural Collaborative Filtering Recommendation System with PyTorch
Introduction
Neural Collaborative Filtering (NCF) is an advanced method for creating recommendation systems. Unlike traditional collaborative filtering techniques that depend on linear models, NCF employs neural networks to understand complex interactions between users and items. This tutorial will guide you through the process of implementing an NCF recommendation system using the MovieLens dataset and PyTorch.
Setup and Environment
To begin, you need to set up your environment by installing the necessary libraries. Use the following command:
pip install torch numpy pandas matplotlib seaborn scikit-learn tqdm
After installation, import the required libraries to prepare your coding environment.
Data Loading and Preparation
We will utilize the MovieLens 100K dataset, which contains 100,000 movie ratings. Here’s how to load and explore the data:
- Download and unzip the dataset.
- Load the ratings and movies data into dataframes.
- Analyze the dataset to understand the number of unique users and items, as well as the distribution of ratings.
Data Preparation for NCF
Prepare the data for the NCF model by splitting it into training and testing sets. Create a custom dataset class to handle user-item interactions efficiently.
Model Architecture
Implement the NCF model, which combines Generalized Matrix Factorization (GMF) and Multi-Layer Perceptron (MLP) components. This architecture allows the model to learn both linear and non-linear relationships between users and items.
Training the Model
Train the NCF model using a binary cross-entropy loss function and the Adam optimizer. Monitor the training process and evaluate the model’s performance on the validation set.
Generating Recommendations
Once the model is trained, create a function to generate personalized recommendations for users based on their preferences. This function will filter out items the user has already rated and provide the top recommendations.
Model Evaluation
Evaluate the model’s effectiveness using various metrics such as AUC (Area Under the Curve) and Average Precision. This will help you understand how well the model performs in predicting user preferences.
Cold Start Analysis
Analyze how the model performs for new users or users with few ratings, addressing the cold start problem. This analysis is crucial for improving the recommendation system’s effectiveness.
Business Insights and Extensions
Consider the following practical business solutions to enhance your recommendation system:
- Integrate hybrid approaches that combine collaborative filtering with content-based filtering.
- Implement attention mechanisms to improve recommendation accuracy.
- Develop deployable web applications to provide real-time recommendations to users.
Conclusion
This tutorial has demonstrated how to implement a Neural Collaborative Filtering recommendation system using PyTorch. By leveraging the MovieLens dataset, we built a model capable of generating personalized content recommendations. The implementation addresses key challenges, including the cold start problem, and provides performance metrics to evaluate effectiveness. This foundational work can be extended to meet various business needs, enhancing user engagement and satisfaction.
For further assistance in managing AI in your business, please contact us at hello@itinai.ru or follow us on our social media platforms.