The article explains the Sobel operator, a kernel used in image processing for edge detection in Convolutional Neural Networks. The operator consists of two kernels for calculating the gradient in the horizontal and vertical directions. It is useful for detecting edges in grayscale images by approximating the gradient of image intensity at each pixel.
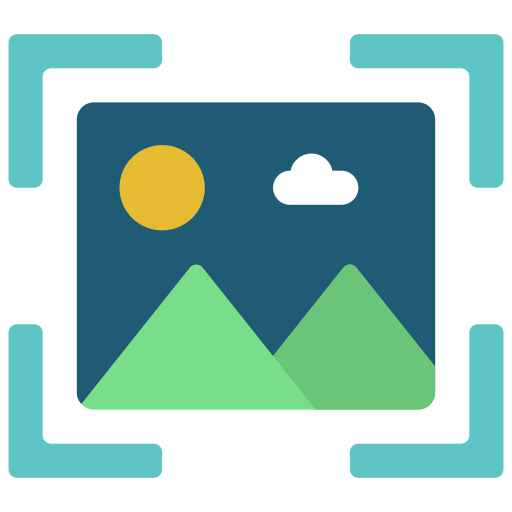
“`html
What is the Sobel operator and how it is used with examples
Image processing icons created by juicy_fish — Flaticon
Convolution Overview
In my previous article, we dived into the key building block behind Convolutional Neural Networks (CNNs), the convolution mathematical operator. I highly recommend you check that out as it builds context and understanding for this article:
Convolution Explained — Introduction to Convolutional Neural Networks
In a nutshell, convolution for image processing is where we apply a small matrix, called a kernel, over our input image to create an output image with some effect applied to it such as blurring or sharpening.
Mathematically, what we have is:
Discrete convolution theorem. Equation by author in LaTeX.
f∗g: Convolution between functions, f and g.
f: The input image
g: The kernel matrix, also known as a filter
t: The pixel where the convolution is being computed.
f(τ): The pixel value of image f at pixel τ.
g(t−τ): The pixel value of g shifted by τ and evaluated at t.
Below is an example of this process, where we apply a box blur to our input image:
Example convolution for applying a blurring effect on a grayscale image. Diagram created by author.
Example of blurring the image. Plot created by author in Python.
The convolution is computed by multiplying each pixel of the input image with the corresponding element from the kernel and summing these products, then normalized by the number of elements.
This is an example of the middle pixel as depicted in the diagram above:
[30*1 + 30*1 + 30*1] +
[30*1 + 70*1 + 30*1] +
[30*1 + 30*1 + 30*1]
= 30 + 30 + 30 + 30 + 70 + 30 + 30 + 30 + 30 = 310
pixel value = 310 / 9 ~ 34
Depending on the structure of the kernel, we get different effects applied to our image. One of the most useful kernels is the Sobel operator, which is going to be the subject of this article!
What is the Sobel Operator?
The Sobel operator is a powerful kernel for edge detection, making it useful for machine learning, particularly Convolutional Neural Networks. It accomplishes this by calculating an approximation of the gradient (first-order derivative) of the image’s intensity at each pixel.
The Sobel operator consists of two kernels, one measures the gradient in the horizontal direction, x, and the other in the vertical direction, y. Mathematically it looks like this:
Sobel Operator. Equation by author in LaTeX.
These kernels are convolved with some input image to calculate the difference in intensity on either side of a pixel we are considering.
Due to their size, 3×3, they are also quick to compute, which is a huge benefit when dealing with deep learning and CNNs. However, this means it has a small resolution, so it may detect edges that humans don’t consider “real” edges.
Images don’t have perfect horizontal or vertical edges, so we need to compute the total magnitude of the gradient. This can be done as follows:
Magnitude of gradient. Equation by author in LaTeX.
There is also a version without the powers that is computationally faster but is an approximation:
Approximation of the gradient magnitude. Equation by author in LaTeX.
We can also calculate the angle of the edge through the use of arctan:
Angle of edge. Equation by author in LaTeX.
It’s important to note that the Sobel operator is only really used for grayscale images as it measures changes in intensity.
Sobel Operator Example
Let’s now walk through an example of applying the Sobel operator to an image.
Below is our grayscale input image, which is 5×5 pixels:
Image:
0 0 0 0 0 0 0 0 0 0 200 200 200 200 200 200 200 200 200 200 200 200 200 200 200
As we can see, the top two rows are all black and the bottom three are white, indicating a vertical edge.
The smaller the pixel value, the darker it is. A value of 0 is black and 255 is white, with values in-between being somewhere on the grayscale.
These are the Sobel operators:
G_x: -1 0 +1 -2 0 +2 -1 0 +1 G_y: -1 -2 -1 0 0 0 +1 +2 +1
Let’s now apply the Sobel operators to the central 3×3 pixel grid of the input image:
Central Grid:
0 0 0 255 255 255 255 255 255
Apply G_x:
(0*-1 + 0*0 + 0*1) +
(255*-2 + 255*0 + 255*2) +
(255*-1 + 255*0 + 255*1) =
0 + 0 + 0 +
-510 + 0 + 510 +
-255 + 0 + 255 = 0
Apply G_y:
(0*-1 + 0*-2 + 0*-1) +
(255*0 + 255*0 + 255*0) +
(255*1 + 255*2 + 255*1) =
0 + 0 + 0 +
0 + 0 + 0 +
255 + 510 + 255 = 1020
G_x is zero, meaning there is no gradient in the horizontal direction, which makes sense when we look at the input images’ pixel values.
The magnitude of the gradient is:
G = (G_x^2 + G_y^2)^0.5 = (0^2 + 1020^2)^0.5 = 1020
And the angle:
theta = arctan(G_y/G_x) = arctan(1020/0) = undefined
As the division by zero is undefined, we can infer the direction is purely in the vertical direction, meaning we have a horizontal edge.
This can all be done in Python as follows:
# Import packages import numpy as np import matplotlib.pyplot as plt from scipy import ndimage # Input image image = np.array([ [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [200, 200, 200, 200, 200], [200, 200, 200, 200, 200], [200, 200, 200, 200, 200] ]) # Sobel Operators G_x = np.array([ [-1, 0, +1], [-2, 0, +2], [-1, 0, +1] ]) G_y = np.array([ [-1, -2, -1], [0, 0, 0], [+1, +2, +1] ]) # Apply the Sobel kernels to the image output_x = ndimage.convolve(image.astype(float), G_x) output_y = ndimage.convolve(image.astype(float), G_y) # Define the light grey color for the background light_grey = [0.8, 0.8, 0.8] # RGB values for light grey # Normalize the Sobel outputs norm_output_x = (output_x - output_x.min()) / (output_x.max() - output_x.min()) norm_output_y = (output_y - output_y.min()) / (output_y.max() - output_y.min()) # Set the background color of the plots plt.rcParams['figure.facecolor'] = light_grey plt.rcParams['axes.facecolor'] = light_grey # Plot the images with the light grey background fig, axes = plt.subplots(1, 3, figsize=(15, 5)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') axes[1].imshow(norm_output_x, cmap='gray') axes[1].set_title('Sobel - Horizontal Direction') axes[1].axis('off') axes[2].imshow(norm_output_y, cmap='gray') axes[2].set_title('Sobel - Vertical Direction') axes[2].axis('off') plt.tight_layout() plt.show()
Sobel operator example. Plot generated by author in Python.
The horizontal Sobel operator is undefined/zero as there is no horizontal edge. Whereas, the vertical Sobel operator had a strong response and detected the area of the edge shown by the black rectangle.
Code available on my GitHub here.
Other Edge Detectors
Sobel is not the only edge detection kernel/algorithm. Below is a list of some other common ones with links for the interested reader:
Canny Edge Detector: Probably the most popular edge detector but has multiple stages. These include a Gaussian blur, differential gradient detection, and suppression.
Prewitt Operator: Very similar to the Sobel operator as it’s also two 3×3 kernels that measure the gradient in intensity in both directions. However, a bit less accurate than the Sobel, but less sensitive to noise.
Robert Cross Operator: Another differential operator but using 2×2 kernels, so a quicker compute time.
Summary & Further Thoughts
In this article, we have shown how you can detect edges in images using the Sobel operator. This operator calculates the discrete approximate gradient in the intensity of an image about a single pixel. If the difference on either side of a pixel is large, it likely lies on an edge. This is the heart of how convolutional neural networks work by finding these types of kernels.
Another Thing!
I have a free newsletter, Dishing the Data, where I share weekly tips for becoming a better Data Scientist, and the latest AI news to keep you in the loop. There is no “fluff” or “clickbait”, just pure actionable insights from a practicing Data Scientist.
Dishing The Data | Egor Howell | Substack
References and Further Reading
Good read on edge detection
Excellent video on Sobel operator
Advice on implementing the Sobel operator
Connect With Me!
YouTube 🎬
Newsletter 📄
LinkedIn 👔
Twitter 🖊
GitHub 🖥
Kaggle 🏅
(All emojis designed by OpenMoji — the open-source emoji and icon project. License: CC BY-SA 4.0)
If you want to evolve your company with AI, stay competitive, use for your advantage Sobel Operator In Image Processing.
Discover how AI can redefine your way of work. Identify Automation Opportunities: Locate key customer interaction points that can benefit from AI.
Define KPIs: Ensure your AI endeavors have measurable impacts on business outcomes.
Select an AI Solution: Choose tools that align with your needs and provide customization.
Implement Gradually: Start with a pilot, gather data, and expand AI usage judiciously.
For AI KPI management advice, connect with us at hello@itinai.com. And for continuous insights into leveraging AI, stay tuned on our Telegram t.me/itinainews or Twitter @itinaicom.
Spotlight on a Practical AI Solution:
Consider the AI Sales Bot from itinai.com/aisalesbot designed to automate customer engagement 24/7 and manage interactions across all customer journey stages.
Discover how AI can redefine your sales processes and customer engagement. Explore solutions at itinai.com.
“`