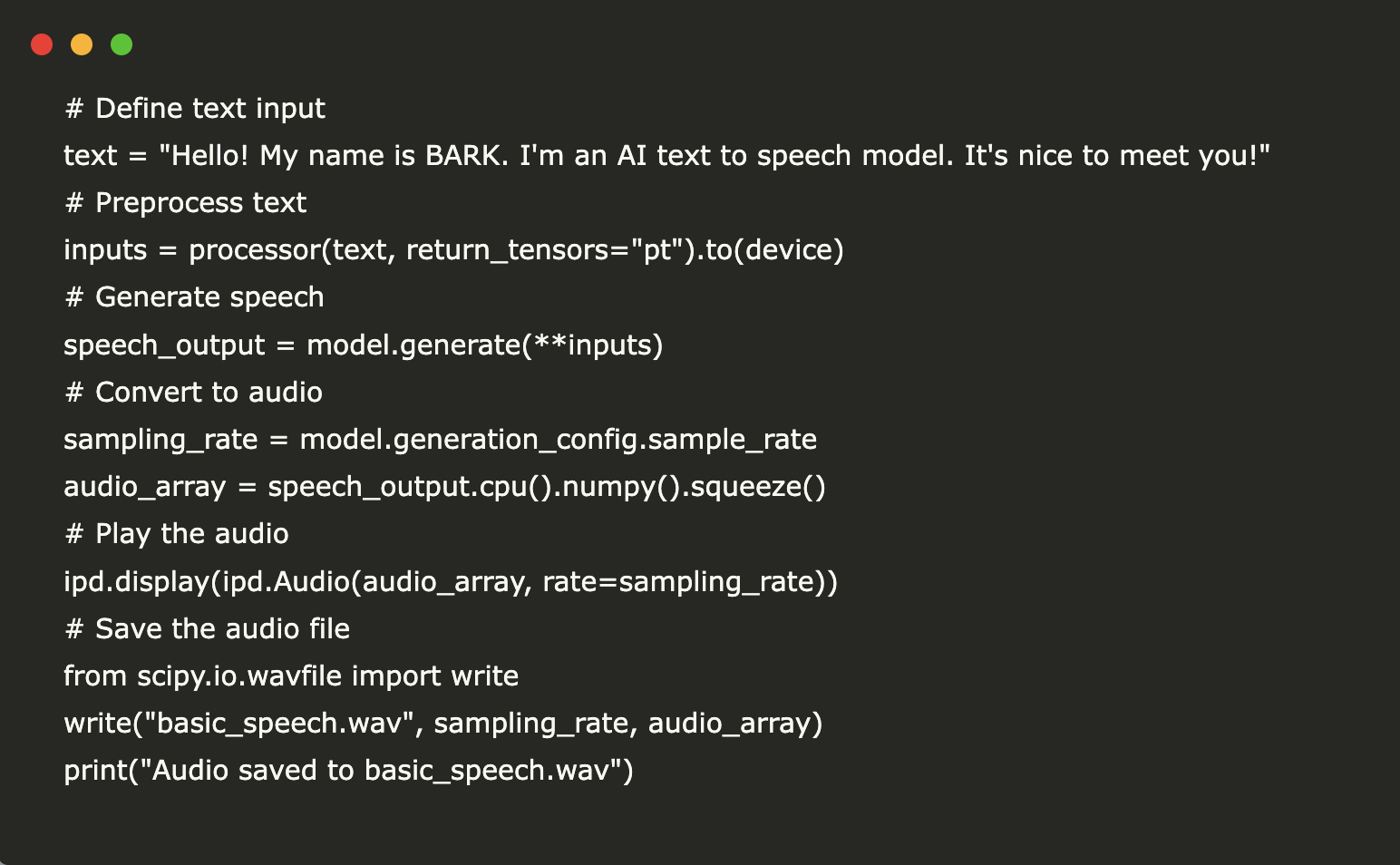
Text-to-Speech Technology Overview
Text-to-Speech (TTS) technology has significantly advanced, evolving from robotic voices to highly natural speech synthesis. BARK, developed by Suno, is an open-source TTS model that generates human-like speech in multiple languages, including non-verbal sounds like laughter and sighs.
Implementation Objectives
In this tutorial, you will learn to:
- Set up and run BARK in Google Colab
- Generate speech from text input
- Experiment with different voices and speaking styles
- Create practical TTS applications
Why BARK is Unique
BARK is a fully generative text-to-audio model capable of producing natural-sounding speech, music, background noise, and sound effects without the need for extensive audio preprocessing or speaker-specific training.
Implementation Steps
Step 1: Setting Up the Environment
Begin by installing the necessary libraries:
!pip install transformers==4.31.0 !pip install accelerate !pip install scipy !pip install torch !pip install torchaudio
Next, import the required libraries:
import torch import numpy as np import IPython.display as ipd from transformers import BarkModel, BarkProcessor
Check if a GPU is available:
device = "cuda" if torch.cuda.is_available() else "cpu" print(f"Using device: {device}")
Step 2: Loading the BARK Model
Load the BARK model and processor:
model = BarkModel.from_pretrained("suno/bark") processor = BarkProcessor.from_pretrained("suno/bark") model = model.to(device)
Step 3: Generating Basic Speech
Generate speech from a simple text example:
text = "Hello! My name is BARK. I'm an AI text to speech model. It's nice to meet you!" inputs = processor(text, return_tensors="pt").to(device) speech_output = model.generate(**inputs) sampling_rate = model.generation_config.sample_rate audio_array = speech_output.cpu().numpy().squeeze() ipd.display(ipd.Audio(audio_array, rate=sampling_rate))
Step 4: Using Different Speaker Presets
Explore predefined speaker presets:
english_speakers = ["v2/en_speaker_0", "v2/en_speaker_1", "v2/en_speaker_2", "v2/en_speaker_3", "v2/en_speaker_4", "v2/en_speaker_5", "v2/en_speaker_6", "v2/en_speaker_7", "v2/en_speaker_8", "v2/en_speaker_9"] speaker = english_speakers[3] text = "BARK can generate speech in different voices." inputs = processor(text, return_tensors="pt", voice_preset=speaker).to(device) speech_output = model.generate(**inputs) audio_array = speech_output.cpu().numpy().squeeze() ipd.display(ipd.Audio(audio_array, rate=sampling_rate))
Step 5: Generating Multilingual Speech
Generate speech in various languages:
texts = { "English": "Hello, how are you doing today?", "Spanish": "¡Hola! ¿Cómo estás hoy?", "French": "Bonjour! Comment allez-vous aujourd'hui?", "German": "Hallo! Wie geht es Ihnen heute?", "Chinese": "你好!今天你好吗?", "Japanese": "こんにちは!今日の調子はどうですか?" } for language, text in texts.items(): voice_preset = None if language == "English": voice_preset = "v2/en_speaker_1" # Additional language presets... inputs = processor(text, return_tensors="pt", voice_preset=voice_preset).to(device) speech_output = model.generate(**inputs) audio_array = speech_output.cpu().numpy().squeeze() ipd.display(ipd.Audio(audio_array, rate=sampling_rate))
Step 6: Creating a Practical Application – Audio Book Generator
Build an audiobook generator that converts text into speech:
def generate_audiobook(text, speaker_preset="v2/en_speaker_2", chunk_size=250): # Function implementation... return full_audio book_excerpt = "Alice was beginning to get very tired..." audiobook = generate_audiobook(book_excerpt) ipd.display(ipd.Audio(audiobook, rate=sampling_rate))
Conclusion
In this tutorial, we have successfully implemented the BARK TTS model using Hugging Face’s Transformers library in Google Colab. Key takeaways include:
- Setting up and loading the BARK model
- Generating basic speech from text
- Using different speaker presets
- Creating multilingual speech
- Building an audiobook generator
Future Experimentation
Consider exploring the following:
- Voice Cloning
- Integration with Other Systems
- Web Application Development
- Custom Fine-tuning
- Performance Optimization
- Quality Evaluation
As you delve deeper into TTS technology, you will uncover more innovative applications and enhancements.
For further assistance or inquiries, please contact us at hello@itinai.ru.
“`