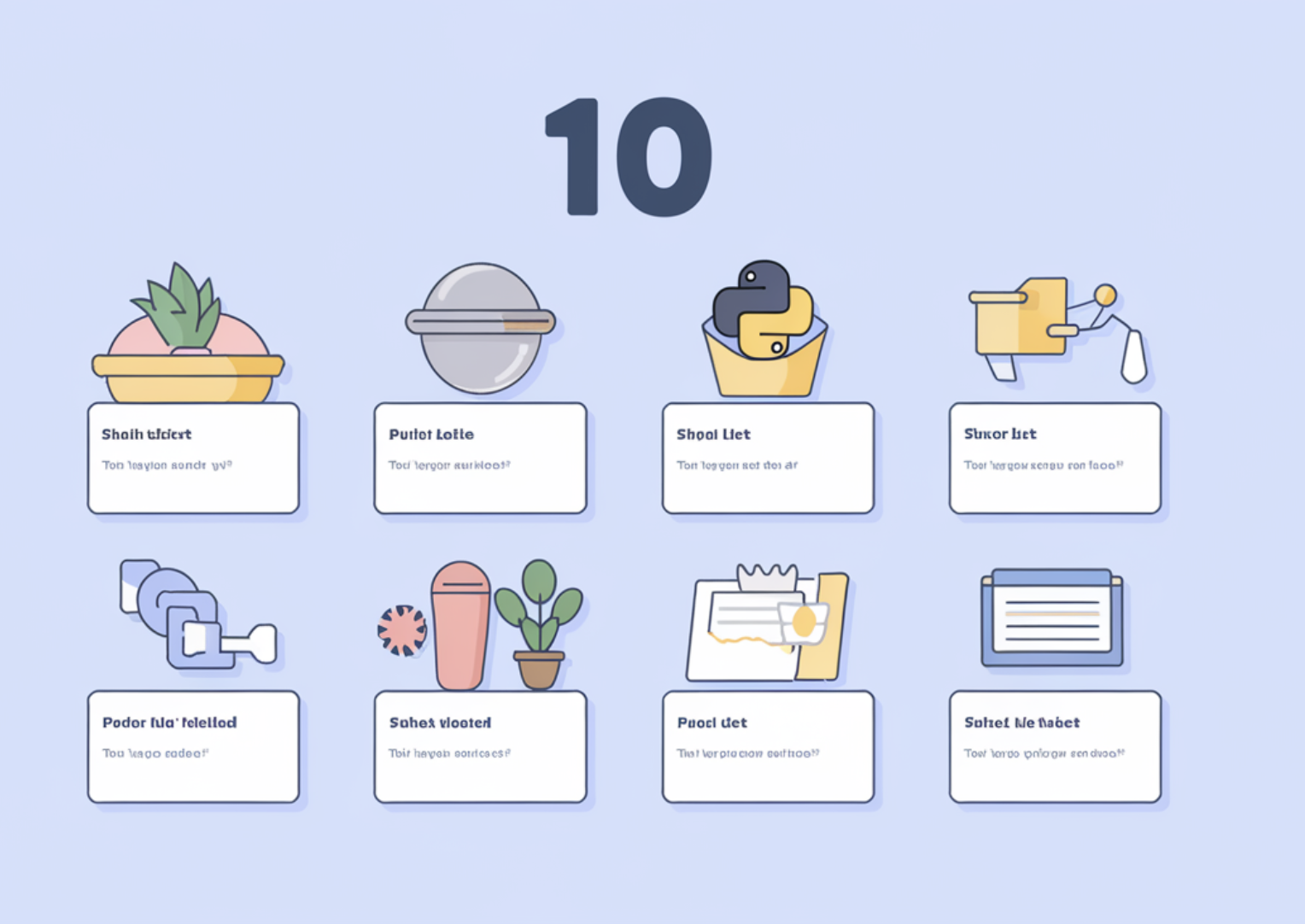
Python’s Filter Function: A Powerful Tool for Data Manipulation
Overview
Python is a flexible programming language that includes effective tools for handling data structures. One of these tools is the filter() function. This function helps to extract elements from a list based on specific criteria, making it essential for tasks like data cleaning and analysis.
10 Effective Methods to Filter Lists in Python
1. Using filter() with a Lambda Function
The filter() function can use a lambda function to define the filtering condition. It processes each element and returns those that meet the condition.
– **Practical Value**: Quick and concise for simple tasks.
– **Complexity**: O(n)
python
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4, 6]
2. Using filter() with a User-Defined Function
Create a custom function to dictate the filtering logic. Useful for more complex conditions.
– **Practical Value**: Clear structure for complicated filtering.
– **Complexity**: O(n)
python
def is_even(x):
return x % 2 == 0
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = list(filter(is_even, numbers))
print(even_numbers) # Output: [2, 4, 6]
3. Using List Comprehension
List comprehensions allow for an elegant way to filter and create new lists based on existing ones.
– **Practical Value**: Streamlined and readable code.
– **Complexity**: O(n)
python
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [x for x in numbers if x % 2 == 0]
print(even_numbers) # Output: [2, 4, 6]
4. Using Generator Expressions
Generator expressions generate items one at a time, which saves memory especially for large datasets.
– **Practical Value**: Efficient for handling big data.
– **Complexity**: O(n)
python
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = (x for x in numbers if x % 2 == 0)
print(list(even_numbers)) # Output: [2, 4, 6]
5. Using NumPyβs where() Function
NumPy’s where() function is ideal for filtering and indexing arrays based on conditions.
– **Practical Value**: Fast and effective for array manipulation.
– **Complexity**: O(n)
python
import numpy as np
numbers = np.array([1, 2, 3, 4, 5, 6])
even_numbers = numbers[np.where(numbers % 2 == 0)]
print(even_numbers) # Output: [2 4 6]
6. Using Pandasβ query() Method
Pandas offers the query() method for filtering DataFrames, making it suitable for larger datasets.
– **Practical Value**: Flexible filtering for complex datasets.
– **Complexity**: Varies by DataFrame size
python
import pandas as pd
df = pd.DataFrame({‘numbers’: [1, 2, 3, 4, 5, 6]})
even_numbers_df = df.query(‘numbers % 2 == 0’)
print(even_numbers_df)
7. Using itertools.filterfalse()
This method filters out elements that do not satisfy the condition, offering an alternative approach.
– **Practical Value**: Useful for retaining non-matching elements.
– **Complexity**: O(n)
python
from itertools import filterfalse
numbers = [1, 2, 3, 4, 5, 6]
odd_numbers = list(filterfalse(lambda x: x % 2 == 0, numbers))
print(odd_numbers) # Output: [1, 3, 5]
8. Using functools.partial()
This method allows for creating partially applied functions to simplify filtering.
– **Practical Value**: Customizable and reusable filter functions.
– **Complexity**: O(n)
python
from functools import partial
def is_divisible_by(x, divisor):
return x % divisor == 0
is_even = partial(is_divisible_by, divisor=2)
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = list(filter(is_even, numbers))
print(even_numbers) # Output: [2, 4, 6]
9. Using a Loop and Conditional
A simple way to filter is by using a loop and condition check.
– **Practical Value**: Easy to understand for beginners.
– **Complexity**: O(n)
python
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = []
for number in numbers:
if number % 2 == 0:
even_numbers.append(number)
print(even_numbers) # Output: [2, 4, 6]
10. Using a Recursive Function
This method applies the filter condition recursively to subsets.
– **Practical Value**: A unique approach for those familiar with recursion.
– **Complexity**: O(n)
python
def filter_recursive(iterable, condition):
if not iterable:
return []
head, *tail = iterable
return [head] + filter_recursive(tail, condition) if condition(head) else filter_recursive(tail, condition)
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = filter_recursive(numbers, lambda x: x % 2 == 0)
print(list(even_numbers)) # Output: [2, 4, 6]
Conclusion
Python’s filter() function and various techniques provide diverse and powerful ways to manipulate data. The choice of method can depend on factors like data format and performance needs. Understanding these methods allows you to effectively analyze and work with data in Python.
Embrace AI for Your Business
To stay competitive, consider implementing the 10 Best Methods to Use Python Filter List. AI can redefine your work processes by:
– **Identifying Automation Opportunities**: Find areas where AI can enhance efficiency.
– **Defining KPIs**: Set measurable goals for your AI initiatives.
– **Selecting Appropriate AI Solutions**: Choose tools tailored to your needs.
– **Gradual Implementation**: Start small, analyze data, and expand wisely.
For AI management advice, contact us at hello@itinai.com. For ongoing insights, follow us on Telegram @itinainews or Twitter @itinaicom.
Experience how AI can transform your sales processes and customer engagement at itinai.com.